Hey there! If you’re diving into the world of React and looking to level up your web development game, you’ve stumbled upon the perfect starting point. Welcome to our “React Router Tutorial,” where we unlock the secrets of navigating the robust yet intriguing world of single-page applications (SPAs) using React Router. So, why wait? Let’s get the ball rolling and discover what makes React Router an indispensable ally in the modern web development arena.
1. Introduction to React Router: Your Gateway to Single-Page Applications Mastery
In the ever-evolving landscape of web development, single-page applications (SPAs) have become the cornerstone of a seamless user experience. Gone are the days of clunky page reloads and disrupted user flows. Thanks to SPAs, users now enjoy smooth, dynamic interactions on websites that feel as responsive as native apps. But, how do we craft these magical experiences? Enter React Router, the conductor of this symphony, ensuring every part of your application is in perfect harmony.
React Router is more than just a tool; it’s your guide through the labyrinth of modern web applications. It’s what turns your React project into a navigable masterpiece, allowing users to move between pages without the page refreshing saga. Think of it as the invisible threads that connect different parts of your app, making navigation seamless and intuitive. Whether you’re building a portfolio, a blog, or an e-commerce site, React Router ensures your users can explore your creation effortlessly.
But why is React Router so pivotal in today’s web development scene? Well, it’s all about enhancing the user experience. With React Router, you create an app that loads a single HTML page and updates the content dynamically as the user interacts with the app. This approach not only makes your app faster and more responsive but also significantly improves its performance. Plus, it’s an excellent way to keep your project organized and maintainable, which is music to any developer’s ears.
Moreover, staying updated with the current version of React Router is crucial. The React Router team is always on the move, introducing new features, fixing bugs, and improving performance. Keeping your skills sharp with the latest version ensures your React applications are not just meeting but exceeding modern web standards.
2. Setting Up React Router
In essence, our journey through this React Router Tutorial will arm you with everything you need to harness the power of React Router. From the basics of setting up routes to the intricacies of nested routes and dynamic routing, we’ve got you covered. So, whether you’re a seasoned developer or just starting, this tutorial is your roadmap to mastering React Router in your next React application. Let’s dive in and explore the wonders of React Router together, shall we?
Step 1: Laying the Foundation with React Router
Before we start, imagine your React app as a bustling city. Now, this city needs roads, signs, and directions to ensure everyone can get to their destinations smoothly, right? React Router does precisely that for your app. It lays down the digital roads and signposts for your users to navigate through your app’s different “locations” without actually leaving the webpage. Neat, huh?
First things first, we need to install React Router. Open up your terminal and run the following command in your project’s root directory:
npm install react-router-dom@6
This magic spell fetches the latest version of React Router from the vast npm library and adds it to your project, giving you all the tools you need to start building your application’s navigation.
Step 2: Setting Up Your First Route
Imagine you’re creating a simple React app—a personal blog, perhaps. You want your visitors to land on a homepage but also navigate to your about page without reloading the entire site. That’s where React Router comes into play.
First, let’s set up our project structure. Inside your src
directory, create two files: HomePage.js
and AboutPage.js
. Think of these as two different areas in your app city. Now, we’ll create simple components for each page:
// HomePage.js
const HomePage = () => <div>Welcome to my blog!</div>;
export default HomePage;
// AboutPage.js
const AboutPage = () => <div>About Me: Here's my story.</div>;
export default AboutPage;
Now, the exciting part—wiring up React Router! Open up your App.js
file. This is where the magic happens:
import React from "react";
import { BrowserRouter, Routes, Route } from "react-router-dom";
import HomePage from "./HomePage";
import AboutPage from "./AboutPage";
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<HomePage />} />
<Route path="/about" element={<AboutPage />} />
</Routes>
</BrowserRouter>
);
}
export default App;
Let’s break down what we just did:
- BrowserRouter: Think of it as the foundation of our city’s road system. It tells our React app to use the HTML5 history API to keep our UI in sync with the URL.
- Routes: This component acts like the city plan, telling our app which roads (routes) lead where.
- Route: Each Route is a road to a different part of our app city. The
path
prop specifies the URL path, and theelement
prop tells the app what to display when the route is matched.
With this setup, navigating to /
shows the HomePage
component, and /about
brings up the AboutPage
. No page reloads, no delays—just smooth transitions between your app’s “locations.”
The Grand Tour
Now, if you start your React app (npm start
), you’ll see the homepage. Add /about
to your URL in the browser, and voilà, you’re on the about page. It’s like teleporting within your app!
What we’ve done here is laid down the first roads in our app city. As you build more features, you’ll add more routes, creating a complex network of navigable paths that enhance your users’ experience.
Remember, React Router is the invisible hand guiding your users through your app’s landscape, making sure they find what they’re looking for with ease and efficiency. And just like city planners ensure a city’s layout is intuitive and user-friendly, you’ll use React Router to craft an application that’s a joy to explore.
So, there you have it—a simple, yet powerful start to using React Router in your React applications. The road ahead is filled with possibilities. Happy coding, explorers!
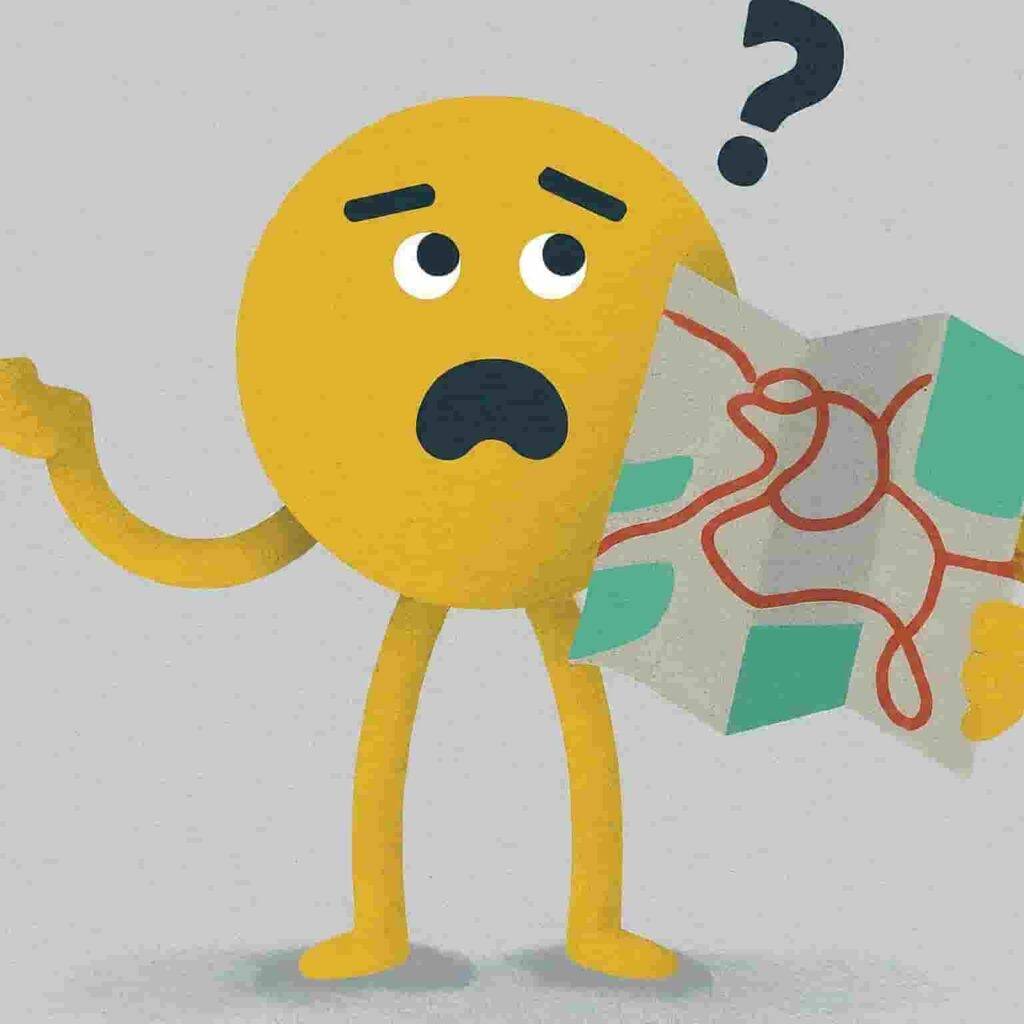
3. Basic Routing Concepts
Imagine you’re crafting a storybook app, where each page tells a different tale. Your readers can magically jump from one tale to another, without flipping through the entire book. This is essentially what we achieve in a React app using React Router. It’s like creating doorways in your app that lead your users exactly where they want to go, with just a click. Today, we’re delving into the heart of React Router – Route components, and how to use the path and element props to create these magical doorways. Plus, we’ll clear up the whole mystery around exact and non-exact paths. So, let’s get this adventure started!
The Essence of Route Components
To bring our storybook app to life, we’ll need some routes. Each route is like a pathway to a different story in our app. React Router uses Route components to define these pathways. Let’s set the scene with a simple example:
Suppose we have two tales to tell: “Home” and “About the Storyteller“. We want our readers to reach these tales through pathways marked /
(our root route) and /about
, respectively. Here’s how we sketch this out:
// In our StorybookApp.js
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import HomePage from './HomePage';
import AboutPage from './AboutPage';
function StorybookApp() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<HomePage />} />
<Route path="/about" element={<AboutPage />} />
</Routes>
</BrowserRouter>
);
}
export default StorybookApp;
- BrowserRouter: Think of it as the enchanted forest encompassing our storybook world. It allows us to use the browser’s history to navigate our app.
- Routes: This is our map of the forest, showing all the pathways (routes) available.
- Route: Each Route is a pathway. The
path
prop marks the trail (URL path), and theelement
prop reveals the story (component) to be shown.
The Magic of path and element Props
- path prop: It’s how you tell the Route which URL should lead to which tale. For example,
path="/about"
means that when our readers navigate to/about
, they want to know more about the storyteller. - element prop: This prop decides what our readers see when they follow a path. By setting
element={<AboutPage />}
, we’re saying that the AboutPage component should display when someone visits/about
.
Unraveling Exact and Non-Exact Paths
In the world of React Router, paths can be exact or non-exact (flexible). Think of exact paths as doors that only open with a specific key. Non-exact paths, however, are more like corridors that lead to multiple doors.
In earlier versions of React Router, you had to specify exact
to match the path precisely. However, with React Router v6, all paths are exact by default. This means if you set a path to /about
, it will only match /about
and not /about/me
or /about/story
. If you want to create nested routes (like having different chapters in a tale), you’ll nest Routes within each other, but that’s a story for another day.
Visualizing Our Routes
Let’s visualize what we’ve done. When a user navigates to /
, they’re greeted with the HomePage component:
Path: /
Output: Welcome to our Storybook!
And when they journey to /about
, the AboutPage component unfolds the tale of the storyteller:
Path: /about
Output: About the Storyteller: Once upon a time...
Just like how every story in a book transports you to a different world, Route components in React Router enable your app to seamlessly transition between views, creating a fluid and intuitive user experience. By understanding the role of path and element props, and the distinction between exact and non-exact paths, you’re well on your way to becoming a master storyteller in the realm of React apps. Happy coding, and may your apps tell the most captivating tales!

4. Dynamic Routing and Parameters
Alright, let’s venture into the realm of dynamic routing, a concept that’s as thrilling as embarking on a treasure hunt where the paths and their destinations change based on the clues you follow. Imagine you’re crafting an online library app. Each book has its own unique story, and similarly, in our app, each book will have its own unique URL. This is where dynamic routing comes into play, allowing us to create flexible paths that adapt to show different book details based on the URL parameters. Let’s set sail on this adventure and see how dynamic routes can bring our library to life.
The Adventure Begins: Setting Up Dynamic Routes
First off, dynamic routes are like secret passages that change their endpoints based on the clues (parameters) you give them. In our library app, we want a way to take our readers directly to the book they’re interested in by using a unique identifier, like the book’s ID, in the URL.
Here’s how we’re going to set this up:
// In our BookApp.js
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import BookList from './BookList';
import BookDetails from './BookDetails';
function BookApp() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<BookList />} />
<Route path="/book/:bookId" element={<BookDetails />} />
</Routes>
</BrowserRouter>
);
}
export default BookApp;
In the above setup:
/book/:bookId
is our dynamic route. The:bookId
part is a placeholder for the actual book ID that will be used in the URL. This is like saying, “Hey, this path will lead to a book’s details, but I’ll tell you exactly which book when you give me its ID.”
Uncovering the Secrets with useParams
Now, how do we get the book’s ID from the URL and display the right book’s details? Enter the useParams
hook, a treasure map that helps us decode the URL parameters.
Let’s explore how to use useParams
to fetch and display the details of a book:
// In our BookDetails.js
import React from 'react';
import { useParams } from 'react-router-dom';
const BookDetails = () => {
let { bookId } = useParams(); // Here's where we access the bookId from the URL
return (
<div>
<h2>Book Details</h2>
<p>Showing details for book ID: {bookId}</p>
{/* In a real app, you'd use bookId to fetch book details from a server */}
</div>
);
}
export default BookDetails;
In BookDetails
, useParams
works like a compass, guiding us to the bookId
hidden in the URL. With this ID, we could fetch more details from a server or a local database, but for now, we’re keeping it simple by just showing the ID.
Charting the Route: Visualizing Our Dynamic Path
When a user navigates to /book/42
, our app dynamically routes them to the BookDetails
component, which then reveals:
Book Details
Showing details for book ID: 42
It’s as if by magic, but really, it’s just the power of dynamic routing at work.
By embracing the magic of dynamic routes and the useParams
hook, we’ve seen how to craft pathways in our app that adapt and lead to content dynamically, based on the clues provided in the URL. This approach not only makes our app more interactive and engaging but also unlocks a chest full of possibilities for creating more personalized and user-centric experiences.
So there you have it, fellow adventurers, a glimpse into the dynamic world of routing with React Router. With these tools in your arsenal, you’re well-equipped to chart a course through the vast seas of dynamic content, creating experiences that are as rich and varied as the stories waiting to be told in our library app. Happy coding, and may your paths always lead to exciting destinations!
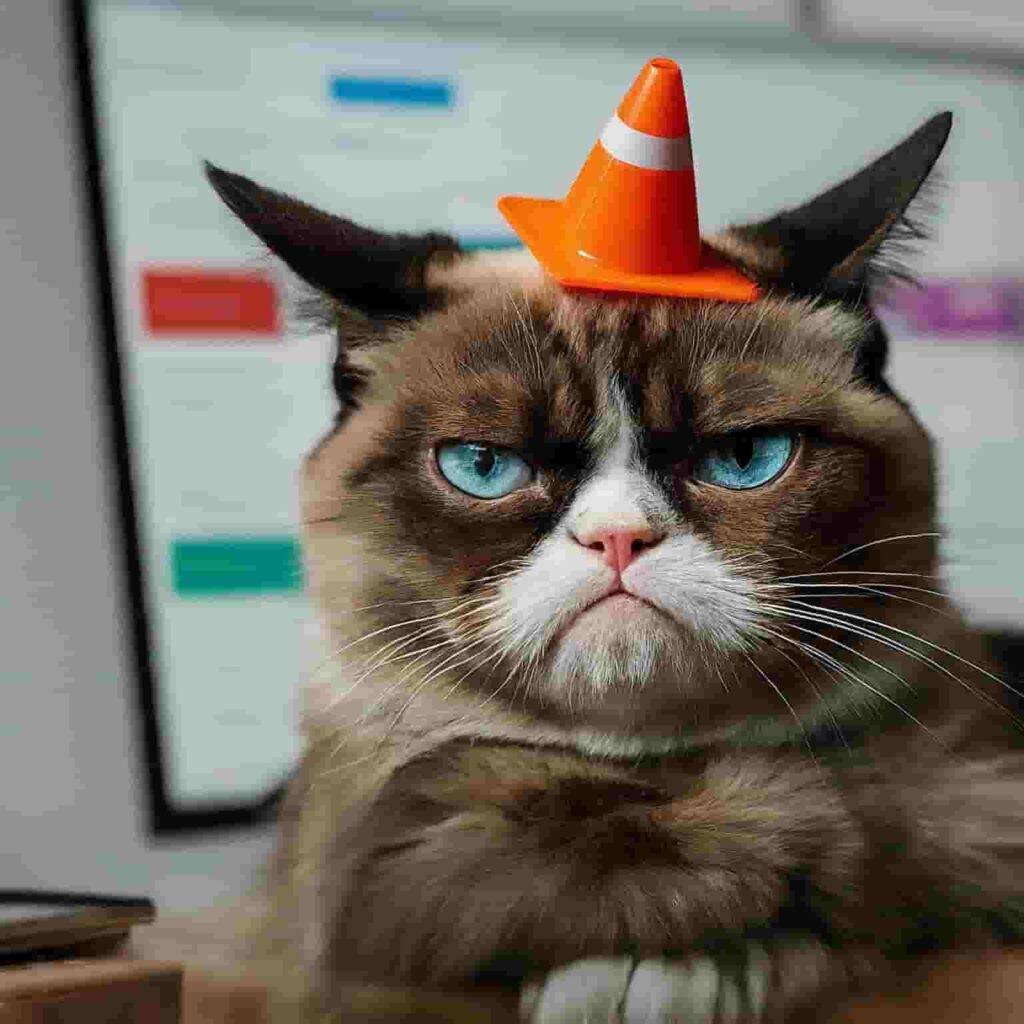
5. Navigation and Links
Gather ’round, folks, as we embark on an adventure through the winding paths of our React app, discovering the magic of navigation. Just like in those fantasy novels where portals transport you to different realms, in React Router, we use something just as magical for navigating our app’s pages: the Link component and the useNavigate
hook. Let’s dive into the hows and whys, shall we?
The Link Component: Our First Portal
First up, let’s talk about the Link component. Imagine you’re creating a website for a cozy little bakery. You’ve got a few pages: Home, About Us, and Contact. Naturally, you want your visitors to move around easily, sampling your offerings without getting lost.
Here’s how we set up our magical portals (a.k.a. navigation links):
// In our BakeryApp.js
import { BrowserRouter, Routes, Route, Link } from 'react-router-dom';
import HomePage from './HomePage';
import AboutPage from './AboutPage';
import ContactPage from './ContactPage';
function BakeryApp() {
return (
<BrowserRouter>
<div>
<nav>
<Link to="/">Home</Link> | <Link to="/about">About Us</Link> | <Link to="/contact">Contact</Link>
</nav>
<Routes>
<Route path="/" element={<HomePage />} />
<Route path="/about" element={<AboutPage />} />
<Route path="/contact" element={<ContactPage />} />
</Routes>
</div>
</BrowserRouter>
);
}
export default BakeryApp;
In this snippet, our Link
components are like enchanting doorways, beckoning your visitors to different sections of your bakery site without the whole page refreshing. Notice how we use to
to specify the destination of each link, creating a seamless, single-page experience that keeps your freshly-baked goodies in front of the customer at all times.
Programmatic Navigation: Steering the Ship with useNavigate
Now, let’s say you want to give a special tour of your bakery through a button click—maybe a surprise page revealing the secret recipe of your world-famous sourdough. This is where useNavigate
comes into play, allowing us to navigate programmatically.
// In our SecretRecipePage.js
import React from 'react';
import { useNavigate } from 'react-router-dom';
const SecretRecipePage = () => {
let navigate = useNavigate();
return (
<div>
<h1>Shh... It's a secret!</h1>
<button onClick={() => navigate('/secret-recipe')}>Discover the Secret Recipe</button>
</div>
);
}
export default SecretRecipePage;
Here, useNavigate
is like having a magical map that takes you exactly where you want to go with just a whisper (or, in our case, a button click). When the visitor clicks the button, they are whisked away to the secret recipe page, no incantations needed.
Visualizing Our useNavigation Hook
Let’s visualize what we’ve accomplished:
- Links in the NavBar:
- Home takes you to the HomePage, displaying all your delightful bakery treats.
- About Us reveals the heartwarming story of your bakery’s journey.
- Contact opens a gateway to conversations over a cup of tea and delicious pastries.
- The Secret Recipe Button:
- Clicking the button in the SecretRecipePage component teleports your visitor to a page where the secret sourdough recipe is revealed, making them feel like part of the bakery family.
Conclusion
In the magical world of React Router, the Link
component and useNavigate
hook are your best friends for navigating the realms of your application. They make the user experience as delightful as walking through a portal into a world of enchantment, or in our case, a cozy, welcoming bakery website. So, go ahead, sprinkle your app with these navigational spells, and watch as your visitors glide smoothly from one page to another, captivated by the magic of your creation. Happy coding, and may your paths always lead to adventurous and engaging user experiences!

6. Nested Routes and Layouts
Ahoy, fellow navigators of the digital sea! Today, we’re charting a course through the intricate archipelago of nested routes in React Router. Just as a matryoshka doll hides smaller dolls within, nested routes allow us to tuck routes within routes, creating a rich, hierarchical structure that mirrors the complex layouts of our applications. Let’s unravel this tapestry, shall we?
The Quest for Nested Routes
Imagine you’re building a mystical academy website. This academy has various departments like Alchemy, Dragon Lore, and Spell Crafting, each with its own subpages. This is a perfect scenario for nested routes: the academy is our parent route, and each department with its subpages are our child routes.
Setting the Stage: Our Magical Academy
Before we delve into the enchantment of nested routes, let’s outline our quest:
- The Academy Portal (Parent Route): This is the main entrance, leading to various departments.
- Departments (Child Routes): Each department has its own realm within the academy, housing further mysteries (subpages).
Crafting Our Enchanted Routes
Let’s create our layout route, which serves as the foundation for our nested routes. This magical framework will host all departmental portals:
// In our AcademyApp.js
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import AcademyLayout from './AcademyLayout';
import Alchemy from './Alchemy';
import DragonLore from './DragonLore';
import SpellCrafting from './SpellCrafting';
function AcademyApp() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<AcademyLayout />}>
<Route index element={<Welcome />} />
<Route path="alchemy" element={<Alchemy />} />
<Route path="dragon-lore" element={<DragonLore />} />
<Route path="spell-crafting" element={<SpellCrafting />} />
</Route>
</Routes>
</BrowserRouter>
);
}
export default AcademyApp;
In AcademyLayout
, we’ve set up a mystical landscape that serves as the foundation. The index
route is our default child route, welcoming wayward travelers. Each department (child route) is nested within, leading to specialized realms of knowledge.
The Magic Unveiled: Understanding Our Enchantment
- AcademyLayout: This is our grand hall, where all paths diverge. It’s the layout route that holds the structure for our nested routes.
- Index Route: Acts as our default child route. It’s the welcoming chamber of the academy, greeting everyone who enters.
- Child Routes: Each department—Alchemy, Dragon Lore, Spell Crafting—has its own sanctum within the academy, accessible through the grand hall.
Behold the Academy!
When a visitor arrives at our academy, they find themselves in the grand hall (AcademyLayout
). From here, they can choose their path:
- / (Root): Displays the
Welcome
component, inviting them to explore further. - /alchemy: Leads to the Alchemy department, revealing secrets of transformation.
- /dragon-lore: Opens the doors to Dragon Lore, where ancient wisdom awaits.
- /spell-crafting: Ushers them into the realm of Spell Crafting, to weave magic with words.
Conclusion
Nested routes in React Router are like exploring a grand, mysterious academy. Each turn reveals new knowledge, hidden rooms, and secret paths. By mastering nested routes, you craft a navigational spell that guides your users through the layers of your app with ease and grace. Whether they’re delving into the depths of Alchemy, soaring through Dragon Lore, or conjuring wonders in Spell Crafting, your app’s architecture supports their journey at every step.
So, intrepid developers, wield the power of nested routes to create complex, yet intuitive, pathways through your applications. May your code be as enchanting as the worlds you build with it. Happy coding, and may your paths always lead to magical destinations!
7. Advanced Routing Features
We’re embarking on an adventure into the heart of React Router’s wilderness, where advanced routing features lie hidden, like ancient ruins awaiting discovery. Our map is marked with intriguing destinations: dynamic segments, custom routes, and the mystical recursive routes. So lace up your boots, and let’s start this expedition!
Unveiling the Mystery: Dynamic Segments
First up, let’s talk about dynamic segments. Imagine you’re creating a social media platform for adventurers where users can share tales of their quests. Each adventurer has a profile page accessible by a unique path, something like /adventurers/:name
. The :name
part is a dynamic segment, changing based on the adventurer’s name. Here’s how we set this up:
// In AdventurerProfiles.js
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import ProfilePage from './ProfilePage';
function AdventurerProfiles() {
return (
<BrowserRouter>
<Routes>
<Route path="/adventurers/:name" element={<ProfilePage />} />
</Routes>
</BrowserRouter>
);
}
And in ProfilePage.js
, we’d use the useParams
hook to access the name
parameter, displaying the correct adventurer’s profile.
Crafting Custom Routes
Now, let’s dive into custom routes. Suppose our adventurer’s platform wants to add a feature where guilds (groups of adventurers) have their unique pages, but not all guilds qualify for a page. We need a route that only renders for guilds that meet specific criteria. This scenario calls for a custom route:
// CustomGuildRoute.js
import { Route, Navigate } from 'react-router-dom';
const CustomGuildRoute = ({ guilds, path, element }) => {
const guildExists = guilds.includes(path.replace('/guilds/', ''));
return guildExists ? <Route path={path} element={element} /> : <Navigate to="/404" />;
};
Here, CustomGuildRoute
checks if the guild exists in our list. If not, the traveler is redirected to a ‘404‘ page. This custom logic ensures only valid guild paths lead to guild pages.
Embarking on the Pathless Journey: Pathless Routes
Imagine a scenario where we want to catch all navigations that don’t match any of our predefined paths and guide them to a specific component, like a mysterious “Forest of Lost Travelers.” This is where pathless routes come into play. They act as a catch-all, ensuring no one gets lost in our app:
// In our RouterSetup.js
<Routes>
{/* Other routes */}
<Route path="*" element={<LostTravelersForest />} />
</Routes>
Anyone who strays off the marked paths ends up in the “Forest of Lost Travelers,” where they can find guidance back to the main trail.
The Lore of Recursive Routes
Lastly, let’s explore the ancient magic of recursive routes. Recursive routes are like dungeons with multiple levels: each level deeper mirrors the structure of the dungeon itself. For our adventurer platform, we might have a recursive route to navigate through a hierarchy of tales, where each tale can contain other tales within it.
Setting up a recursive route requires a bit of ingenuity, as the component needs to know how to render itself when it encounters sub-tales:
// TaleComponent.js
const TaleComponent = ({ tales }) => {
return (
<div>
{tales.map(tale => (
<div key={tale.id}>
<h3>{tale.title}</h3>
{/* Render TaleComponent recursively for sub-tales */}
{tale.subTales && <TaleComponent tales={tale.subTales} />}
</div>
))}
</div>
);
};
This recursive pattern allows for a deeply nested structure, perfect for representing a complex hierarchy of tales and adventures.
Charting Our Discoveries
Our journey through the advanced features of React Router reveals the flexibility and power at our disposal. Dynamic segments allow us to tailor our app’s navigation to the individual, custom routes enable us to build logic directly into our routing, pathless routes catch any wanderers, and recursive routes let us construct complex navigational hierarchies.
As we conclude today’s expedition, remember that these advanced routing techniques are like secret passages in the vast castle of React Router, each leading to new realms of possibilities. With these tools, you’re well-equipped to build navigation structures as intricate and fascinating as the adventures they represent. Happy coding, and may your paths always lead you to exciting new discoveries!
8. Hooks in React Router
We’re venturing into the heart of React Router once more, but this time, we’re focusing on some of its most versatile tools: hooks. Specifically, we’ll explore useLocation
, useState
, and how they can work together to supercharge your app’s navigation and state management. Let’s imagine we’re creating an app for a grand library, one filled with countless books and secret passages (routes). Our goal? To help users find their way and keep track of their journey through this maze of knowledge.
Navigating the Library: The useLocation
Hook
First up, let’s talk about useLocation
. This hook is like a map you find at the entrance of our grand library. It tells you exactly where you are, allowing you to understand your current context within the labyrinth of bookshelves (or, in our case, routes).
Here’s how we can use useLocation
to show breadcrumbs on our library app. Breadcrumbs are a great way to show users their current location and how they got there, enhancing their navigational experience:
import { useLocation } from 'react-router-dom';
const Breadcrumbs = () => {
let location = useLocation();
let paths = location.pathname.split('/').filter(x => x);
return (
<div>
<p>You are here:</p>
<ul>
{paths.map((path, index) => (
<li key={index}>{path}</li>
))}
</ul>
</div>
);
};
In this snippet, useLocation
gives us the current URL’s path, which we then split and filter to create a simple breadcrumb trail. Users can see how deep they’ve ventured into our library, from “Home” to “Fantasy” to “Tales of the Ancient Dragons”, for instance.
Remembering Our Steps: The useState
Hook
Now, let’s pair our location-aware breadcrumbs with the ability to remember and display the last few books a user has viewed. For this, we’ll employ the useState
hook, a magical tome that allows us to keep track of state within our components.
import { useState } from 'react';
import { Link, useNavigate, useLocation } from 'react-router-dom';
const BookHistory = () => {
let [history, setHistory] = useState([]);
let navigate = useNavigate();
let location = useLocation();
// When the location changes, add the new book to our history
useEffect(() => {
setHistory(prevHistory => [...prevHistory, location.pathname]);
}, [location]);
return (
<div>
<h3>Your Book Trail:</h3>
<ul>
{history.map((path, index) => (
<li key={index}>
<Link to={path}>{path}</Link>
</li>
))}
</ul>
<button onClick={() => navigate(-1)}>Go Back</button>
</div>
);
};
In our BookHistory
component, we use useState
to maintain a list of books (routes) the user has visited. Every time the user navigates to a new book, useLocation
detects the change, and we update our history with the new path. We also include a handy “Go Back” button, using useNavigate
to allow users to retrace their steps—a crucial feature in any large library.
Visualizing Our Journey
Let’s visualize what happens as a user navigates through our app:
- Breadcrumbs:
- Home > Fantasy > Tales of the Ancient Dragons
- Book History:
- /home
- /fantasy
- /fantasy/tales-of-the-ancient-dragons
With each step, our breadcrumbs and book history update, providing the user with a clear picture of their journey through our library and the means to navigate it effortlessly.
By leveraging React Router’s hooks, like useLocation
and useState
, alongside navigational aids like useNavigate
, we’ve created a library app that not only guides users through its vast collection but remembers where they’ve been, enhancing their experience and engagement. These hooks are the magical ingredients that make our library not just a collection of books but a navigable world of stories waiting to be explored.
So there you have it, dear developers. With these tools in your belt, you’re well-equipped to build React apps that are as easy to navigate as they are engaging. Happy coding, and may your users always find their way!
9. Handling Authentication and Protected Routes
Welcome to the part of our journey where we fortify our castle gates and ensure that only the rightful knights and ladies can access the treasures within. That’s right; we’re talking about safeguarding our routes in React applications. Imagine you’re creating a realm (app) where secrets (content) should only be revealed to those who have proven their allegiance (logged in users). Let’s embark on this noble quest together, learning how to manage authentication and implement protected routes in our kingdom.
The Royal Decree: Authentication Status
First, we must establish a way to recognize our allies. This is where the concept of authentication status comes into play. Think of it as a royal seal—without it, no one can pass through the gates of our castle (protected routes). We’ll use a simple flag, isAuthenticated
, to keep track of whether a user has been granted the royal seal.
The Guard at the Gate: Creating a Protected Route
Our next step is to station a loyal guard at the gate who checks for the royal seal before allowing anyone through. In React Router terms, this guard is a custom component that wraps around our routes, ensuring that only authenticated users can access them.
Let’s create this component, shall we?
import { Navigate, Outlet } from 'react-router-dom';
const ProtectedRoute = ({ isAuthenticated }) => {
return isAuthenticated ? <Outlet /> : <Navigate to="/login" />;
};
In this component:
<Outlet />
acts as a placeholder that renders the child routes if the user is authenticated.<Navigate to="/login" />
redirects unauthenticated users to the login page, kindly asking them to prove their allegiance.
Fortifying Our Routes
Now, let’s see how to use our ProtectedRoute
to secure the royal library, a place where only the wise and worthy should venture.
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import ProtectedRoute from './ProtectedRoute';
import Library from './Library';
import Login from './Login';
function App() {
const isAuthenticated = /* Logic to determine if user is authenticated */;
return (
<BrowserRouter>
<Routes>
<Route path="/login" element={<Login />} />
<Route element={<ProtectedRoute isAuthenticated={isAuthenticated} />}>
<Route path="/library" element={<Library />} />
{/* Add more protected routes here */}
</Route>
</Routes>
</BrowserRouter>
);
}
In our kingdom, the /library
is now a sanctuary protected by our loyal guard. Only those who have proven their allegiance (by logging in) can gaze upon the ancient tomes and scrolls.
Visualizing the Castle’s Defenses
Let’s visualize what happens when a friend and a foe approach our castle:
- Friend (Authenticated User):
- Tries to access
/library
- The guard checks and sees the royal seal (user is authenticated)
- The gates open, and the user is allowed to enter the library
- Tries to access
- Foe (Unauthenticated User):
- Tries to access
/library
- The guard checks and finds no royal seal (user is not authenticated)
- The foe is redirected to
/login
to prove their allegiance
- Tries to access
By setting up a simple yet effective authentication check and protecting our routes, we ensure that our app’s sensitive information remains secure behind our castle walls. Only those who have the royal seal can access the treasures within, keeping the realm safe and content in the right hands.
So there you have it, brave developers. With these strategies for managing authentication and securing routes, your React kingdom is well-defended against invaders, and peace reigns in the land. Now, go forth and build, knowing your realms are safe!
10. Performance and Optimization
Where the need for speed meets the craft of routing. Today, we’re tuning our engines (apps) to ensure they zoom across the digital highway, offering our users the smoothest and fastest ride possible. Let’s dive into some performance optimization techniques for our client-side routing that not only enhance user experience but also ensure our app doesn’t turn into a gas-guzzler that slows down the journey.
The Pit Stop: Why Optimize?
Imagine our app as a race car in a grand prix of user satisfaction. Every millisecond counts. In the world of client-side routing, every unnecessary reroute or delay is like adding extra weight to our car, slowing us down. Optimizing our routing ensures we’re only carrying what we need, making sharp turns (transitions between views) effortlessly and keeping our users glued to their seats with excitement.
Turbocharging the Ride: Lazy Loading
Our first tip is like giving our car a turbo boost. In technical terms, it’s called “Lazy Loading.” This means our app only loads the content necessary for the current route, rather than carrying the weight of the entire app on each page load.
Here’s how we implement it:
import { lazy, Suspense } from 'react';
import { BrowserRouter, Routes, Route } from 'react-router-dom';
const HomePage = lazy(() => import('./HomePage'));
const AboutPage = lazy(() => import('./AboutPage'));
function App() {
return (
<BrowserRouter>
<Suspense fallback={<div>Loading...</div>}>
<Routes>
<Route path="/" element={<HomePage />} />
<Route path="/about" element={<AboutPage />} />
</Routes>
</Suspense>
</BrowserRouter>
);
}
In this setup, HomePage
and AboutPage
are only loaded when they’re needed, not before. It’s like having extra engines that only turn on when you decide to accelerate, keeping your initial load time lightning fast.
Keeping the Engine Cool: Code Splitting
Code splitting goes hand in hand with lazy loading. It involves splitting your app’s code into smaller chunks, which can then be loaded on demand. This way, users only download the parts of your app necessary for what they’re currently viewing, rather than the entire application upfront.
Webpack, a popular module bundler, comes with built-in support for code splitting. Using dynamic import()
syntax is a common approach to achieving this:
// Before: Single chunk
import { HomePage, AboutPage } from './Pages';
// After: Split into separate chunks
const HomePage = lazy(() => import('./HomePage'));
const AboutPage = lazy(() => import('./AboutPage'));
Fine-Tuning with Prefetching
Prefetching is like planning your pit stops ahead in the race. By prefetching data for your next routes in advance, you ensure that when the user decides to visit them, the content loads almost instantaneously. React Router doesn’t handle data fetching directly, but you can combine it with other libraries or custom logic to prefetch data as soon as certain routes become likely navigation targets.
// Example of prefetching data for a route
// Assume fetchDataForAboutPage is a function that fetches data required by the AboutPage component
const prefetchAboutPageData = () => {
if (/* logic to determine if the user is likely to navigate to AboutPage */) {
fetchDataForAboutPage();
}
};
Smoothing the Corners: Effective Caching
Effective caching strategies ensure that once data or resources are loaded, they’re stored close at hand for future access, eliminating the need for repeated requests. Using service workers, browser caching, and strategic cache headers can significantly improve the performance of your client-side routing.
The Victory Lap
Optimizing client-side routing is not just about making your app faster; it’s about enhancing the overall user experience. It’s the difference between a smooth, enjoyable ride and one that’s bogged down by delays and loading screens. By implementing lazy loading, code splitting, prefetching, and effective caching, you’re not just tuning your app for speed; you’re also preparing it for the long haul, ensuring it remains responsive and efficient no matter how complex it grows.
So, rev those engines, and let’s make our apps not only the fastest on the track but also the most exhilarating to navigate. Happy coding, and may your routes always lead to optimized performance and delighted users!
11. Troubleshooting and Advanced Techniques
Ah, the final frontier in our React Router journey: the shadowy realm of troubleshooting and advanced navigation techniques. Here, we arm ourselves with the torch of knowledge to illuminate the path through the darker, more intricate corridors of React Router. Let’s decode the mystic scripts (documentation) and conjure solutions to common enchantments (challenges) that might bewitch our applications.
Deciphering the Ancient Scrolls: Router Documentation
First and foremost, the Router documentation is like an ancient tome filled with spells (solutions) for almost every conceivable challenge you might face in the realm of routing. Whether you’re a novice wizard just starting out or an experienced mage, the documentation holds the answers to harnessing the full power of React Router. It covers everything from basic route configuration to handling complex scenarios with grace.
The Rite of Passage: Accessing Route Props
A common challenge you might encounter is accessing route-related properties, such as parameters from the URL or the current location. React Router v6 introduces hooks like useParams
and useLocation
to make this task easier than ever.
import { useParams, useLocation } from 'react-router-dom';
const PotionDetails = () => {
let { potionId } = useParams();
let location = useLocation();
// Now you have access to potionId and the current location
console.log("Brewing details for potion:", potionId, "at", location.pathname);
return <div>Details about potion {potionId}</div>;
};
Conjuring Dynamic Pathways: Array of Routes
Sometimes, you need to create routes dynamically, for instance, based on data fetched from a distant land (server). Fear not, for React Router allows you to construct an array of routes, making your application as flexible as a willow in the wind.
const routes = [
{ path: '/potion/:potionId', element: <PotionDetails /> },
// More routes here
];
return (
<Routes>
{routes.map((route, index) => (
<Route key={index} path={route.path} element={route.element} />
))}
</Routes>
);
Vanquishing the Shadows: Destroying Routes
In some tales, there comes a time to close a path forever, to “destroy” a route. While React Router doesn’t have a “destroy route” spell per se, you can achieve this by simply removing the route from your route configuration or toggling its presence based on your application’s state.
Advanced Enchantments: Route Action and Route Config
For those seeking to perform more advanced magic, like executing actions when a route is activated or configuring routes outside of the JSX realm, React Router’s use of hooks and context provides the flexibility needed. While v6 focuses on simplifying route configuration, there are patterns you can follow to add custom logic to your routes:
const useRouteAction = (action) => {
let navigate = useNavigate();
useEffect(() => {
action();
// Example action: navigate back
navigate(-1);
}, [action, navigate]);
};
// Then, in your component:
useRouteAction(() => console.log("This potion is powerful!"));
The Alchemy of Troubleshooting
When you find yourself entangled in thorns, remember, the React Router documentation is your grimoire, your source of ancient wisdom. It’s also wise to join communities of fellow wizards (developers) where you can share tales of adventure and learn from each other’s quests.
In Closing
As we draw the curtains on our epic saga through the lands of React Router, remember that the path of a developer is both challenging and rewarding. Armed with the knowledge of troubleshooting and advanced techniques, you’re now equipped to navigate through the darkest forests and the deepest dungeons of routing in your applications. May your journey be filled with discovery, and may your applications guide your users on many splendid adventures. Happy coding, brave traveler!
12. Conclusion and Further Exploration
As we bring our “React Router Tutorial” voyage to a close, let’s take a moment to look back at the ground we’ve covered. From the bustling crossroads of basic routing to the hidden pathways of advanced techniques, we’ve journeyed through the heart of React Router v6, uncovering its secrets and mastering its spells. Our adventure has taken us through enchanted forests of dynamic routes, over mountains of nested routes, and into the depths of performance optimization—all to ensure that the realms (apps) we build are as navigable as they are mesmerizing.
The Scrolls of Knowledge: A Recap
- Setting the Stage: We began by laying the foundation stones with React Router, ensuring our applications were ready for the journey ahead.
- Navigating the Pathways: We learned how to create and navigate routes, transforming our apps into sprawling cities, each path leading to new discoveries.
- Guarding the Gates: We stood watch at the castle gates, implementing authentication to protect the treasures within.
- Enhancing the Journey: We then turned our attention to performance, optimizing our routes for speed and efficiency, ensuring a seamless voyage for our users.
Beyond the Horizon: Further Exploration
But, as with any great adventure, there is always more to explore. The lands of React Router v6 are vast and filled with untold mysteries. Advanced React developers will find that their exploration of routing approaches and popular routing implementations can lead to innovative solutions and new user experiences.
- Dive Deeper into React Router v6: The latest version of React Router offers a plethora of advanced features and hooks designed to give you greater control over your routing logic. From the powerful
useRoutes
hook to the flexibility of nested routes, there’s a wealth of knowledge waiting to be discovered. - Experiment with Advanced Routing Patterns: Consider how you might use React Router to solve complex navigation scenarios. What unique user experiences can you create by combining nested routes, dynamic segments, and custom hooks?
- Contribute to the Community: The React community thrives on collaboration and shared knowledge. Consider contributing to open-source projects, writing about your discoveries, or creating tutorials to help guide fellow adventurers.
- Stay Informed: The world of web development is ever-changing. Keep your knowledge fresh by staying up-to-date with the latest releases and updates from React Router and the wider React ecosystem.
The End of One Journey Is the Beginning of Another
Our “React Router Tutorial” may have reached its end, but your journey as a developer is just beginning. Armed with the knowledge and skills you’ve acquired, you’re now equipped to tackle new challenges and build even more amazing applications.
Remember, the path of learning is winding and full of surprises. Embrace the exploration, enjoy the process, and let your curiosity guide you to new horizons. The realms of React and React Router are rich with possibilities—dare to dream big, and let your adventures in routing begin!
Happy coding, fellow travelers! May your paths be clear, your applications robust, and your user experiences delightful. Onward to new adventures!
Pingback: What's New in React Router v6? - CodeWithNazam
Pingback: Dynamic Routing in React Router - CodeWithNazam